The problem with Flow Designer outputs
Flow Designer is ServiceNow’s latest workflow engine, replacing the legacy “Workflow”. As I’ve started to build more Flows, one issue I’ve encountered is that viewing the output of flows, subflows and flow actions is often truncated in the GUI. This truncation is generally not a problem when flows execute successfully, as I’m often using or writing that output somewhere else where I can read it if necessary. Here is an example truncated output of a flow action that generates a string containing the numbers from 1 to 10,000:
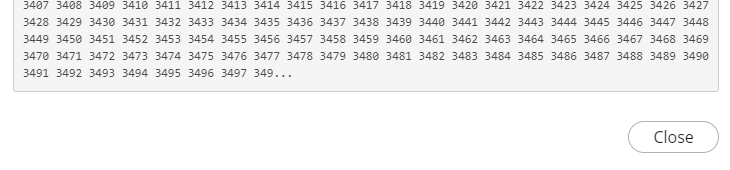
However, I’ve experienced cases where flow actions have generated significant output and then errored. This causes the flow to halt and not proceed to the next step. This means that the output of that action was not saved to the normal location and the flow action output is too long to view in the GUI. But what if I need to see the end of the output to understand why the action failed?
This problem is particularly bad in Powershell steps within flow actions, as they can ‘Error’ even when the command was successful. That means you can’t even read the errors in the next flow action step, because the flow just stops. I’ve learned to always wrap Powershell steps in a try…catch block and handle errors explicitly.
How to view the full output of Flow Designer actions
The output of flow actions (along with other meta-data about the execution) is stored in sys_json_chunk. You might notice that this table has 1-n entries for each flow action step, in a particular ‘position’. You might notice that the ‘data’ field on this table look like it’s Base64 encoded.
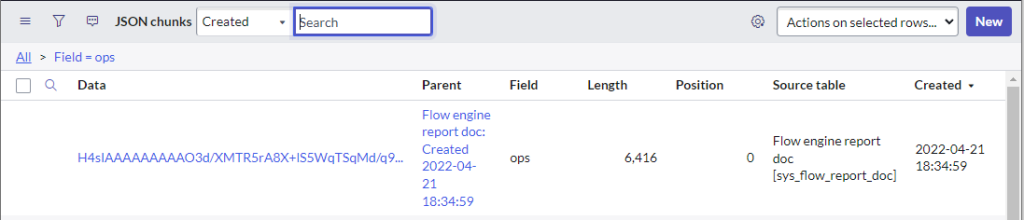
But if you decode that data, it’s unicode gibberish:
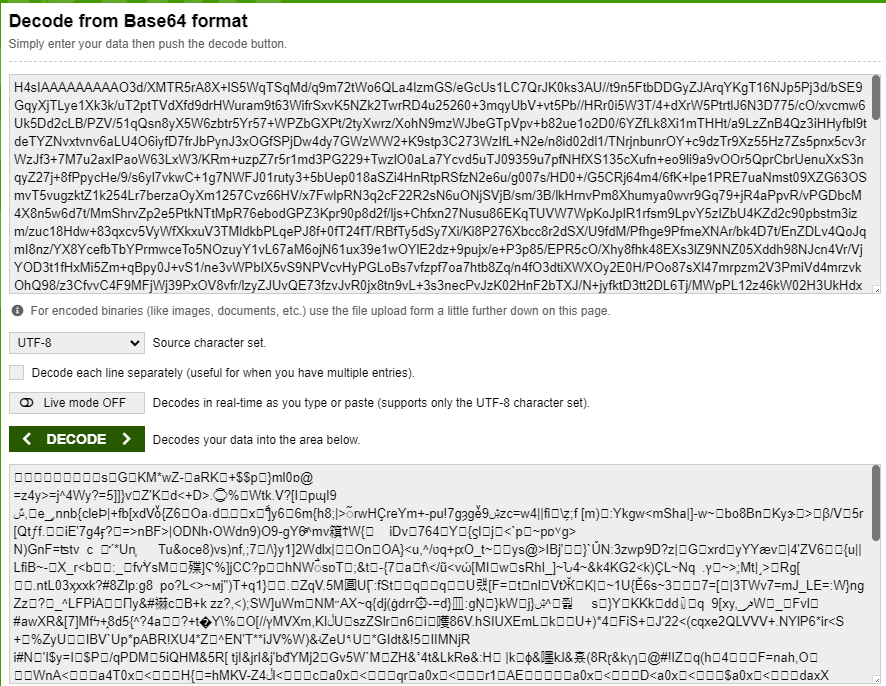
It turns out that this data is simply the compressed flow output, encoded in Base64 and split across 1-n entries in sys_json_chunk. Here is an example of two chunks:

To get at the content of these chunks, luckily ServiceNow has a library to compress and de-compress data, as well as libraries to encode and decode Base64. So to decode a single sys_json_chunk:
var chunk = new GlideRecord('sys_json_chunk');
chunk.get('sys_id_of_sys_json_chunk');
var cs = GlideStringUtil.base64DecodeAsBytes(chunk.data);
gs.print(String(GlideCompressionUtil.expandToString(cs)));
And then if you want to retrieve the full output of a flow action:
- Retrieve the sys_json_chunks based on the sys_id of the parent execution (document_id), in ‘position’ order.
- Base64 decode each data field
- Decompress each data field
- Concatenate the resulting strings together
Here is an example that does everything besides concatenate the final strings, so you can see where they break apart:
var chunk = new GlideRecord('sys_json_chunk');
chunk.addQuery('document_id','21c4c88ddb4701108c1cb7e8f496194a');
chunk.orderBy('position')
chunk.query();
while (chunk.next())
{
var cs = GlideStringUtil.base64DecodeAsBytes(chunk.data);
gs.print(String(GlideCompressionUtil.expandToString(cs)));
}
This will generate an output like:

Generally the output of a flow or an action will be in the sys_json_chunk table, with a source table of “sys_flow_runtime_value” and a field of “output”, or it will have a source table of “sys_flow_report_doc” and a field value of “ops”.